Before you refer this blog, refer this blogpost
https://santoshpoojari.blogspot.com.au/2017/03/sitecore-active-directorysitecoreadmemb.html
I have taken more generic approach to add this. I have refined the above method with c# generic constraint.
Things to be taken care.
We cannot use below Providers add due: Exception Details: System.NotSupportedException: Collection is read-only.
//Membership.Providers.Add(provider);
Therefore we should use utility class that give extension method AddTo.
http://stackoverflow.com/questions/1432508/programmatically-adding-a-membership-provider
Generic Membership Provider
public static void InitialiseProviders <T>(T provider, string providerName, NameValueCollection providerConfiguration) where T : MembershipProvider
{
provider.Initialize(providerName, providerConfiguration);
provider.AddTo(Membership.Providers);
//We cannot use below Providers add due: Exception Details: System.NotSupportedException: Collection is read-only.
//Membership.Providers.Add(provider);
}
Utility Extension Method
public static class ProviderUtil
{
static private FieldInfo providerCollectionReadOnlyField;
static ProviderUtil()
{
Type t = typeof(ProviderCollection);
providerCollectionReadOnlyField = t.GetField("_ReadOnly", BindingFlags.Instance | BindingFlags.NonPublic);
}
static public void AddTo(this ProviderBase provider, ProviderCollection pc)
{
bool prevValue = (bool)providerCollectionReadOnlyField.GetValue(pc);
if (prevValue)
providerCollectionReadOnlyField.SetValue(pc, false);
pc.Add(provider);
if (prevValue)
providerCollectionReadOnlyField.SetValue(pc, true);
}
}
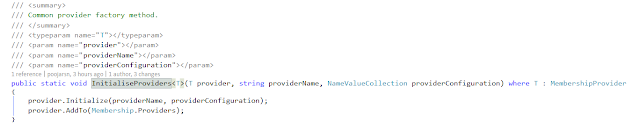
Initialize the LDap AD Configuration and call the generic method
public static NameValueCollection LdapSitecoreProviderConfiguration
{
get {
NameValueCollection config = new NameValueCollection();
config["type"] = "LightLDAP.SitecoreADMembershipProvider";
config["name"] = "SomeProviderName";
config["connectionStringName"] = "LDAPConnString";
config["applicationName"] = "sitecore";
config["connectionUsername"] = ConfigurationManager.AppSettings["AppSettingKeys-UserName"];
config["connectionPassword"] = ConfigurationManager.AppSettings["AppSettingKeys-Password"];
config["minRequiredPasswordLength"] = "1";
config["minRequiredNonalphanumericCharacters"] = "0";
config["requiresQuestionAndAnswer"] = "false";
config["requiresUniqueEmail"] = "false";
config["connectionProtection"] = "secure";
config["attributeMapUsername"] = "sAMAccountName";
config["enableSearchMethods"] = "true";
config["customFilter"] = "(memberOf=Your org custom filter)";
return config;
}
}
InitialiseProviders(new SitecoreADMembershipProvider(), "someldapName", xyz.LdapSitecoreProviderConfiguration);